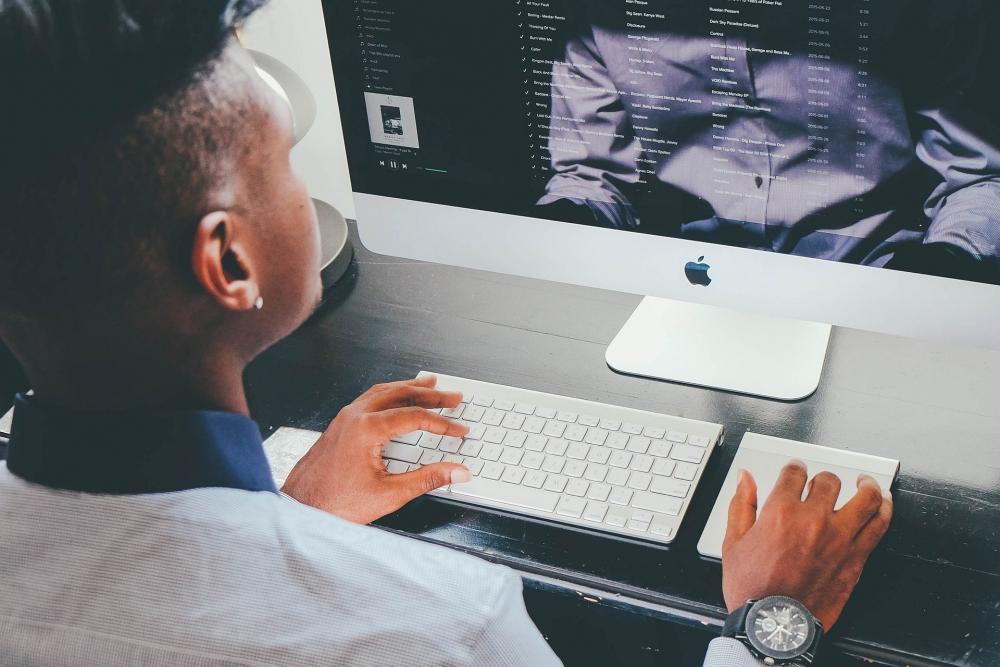
Computer Science Coursework Example
Sorting algorithms serve as fundamental tools for organizing data in computing. They are pivotal in various applications, ranging from simple list arrangements to complex data processing tasks. Consider a scenario where a large dataset needs to be organized in ascending order for efficient searching or analysis. Sorting algorithms determine how quickly and effectively this can be accomplished. Algorithm efficiency, specifically in sorting, measures the ability of these algorithms to perform these tasks swiftly and with minimal resource consumption.
Sorting Algorithms Overview:
- Bubble Sort: This algorithm repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. For instance, arranging a sequence of numbers [5, 2, 9, 1, 5] using Bubble Sort would involve multiple passes until the list is sorted.
- Merge Sort: It divides the list into smaller parts, sorts them individually, and then merges them back together. For instance, splitting [4, 7, 2, 5] into smaller parts [4, 7] and [2, 5], sorting them, and merging them would result in the sorted list [2, 4, 5, 7].
- Quick Sort: This algorithm selects a 'pivot' element and partitions the list into smaller elements based on the pivot, then recursively sorts the partitions. For instance, sorting [3, 9, 1, 7, 5] involves selecting a pivot (let's say 5), partitioning the list into smaller elements less than 5 and greater than 5, and recursively sorting those partitions.
- Selection Sort: It repeatedly selects the minimum element from an unsorted portion and moves it to the sorted portion of the list. For instance, sorting [8, 3, 5, 4, 6] involves finding the minimum element (3), moving it to the beginning, and repeating the process.
- Insertion Sort: This algorithm builds the final sorted list one element at a time by inserting elements into their correct positions. For example, sorting [12, 11, 13, 5, 6] involves inserting each element in its proper place in the growing sorted list.
Comparative Analysis:
- Time Complexity: A measure of the time taken by an algorithm to complete as a function of the input size.
- Space Complexity: Refers to the amount of memory space an algorithm requires to complete its task.
- Best, Average, and Worst-Case Scenarios: Describes the performance of algorithms under different input conditions, such as already sorted, random, or reverse-sorted lists.
Selection and Experiment Setup:
- Programming Language: Selection of a programming language (e.g., Python, Java) for algorithm implementation.
- Test Cases and Datasets: Creating datasets that vary in size and complexity to assess algorithm performance comprehensively.
- Tools and Software: Utilization of analysis tools, such as Python libraries or specific software, for precise performance measurement.
Performance Metrics:
- Execution Time: Measuring the time taken by each algorithm to complete its sorting task for varying datasets.
- Memory Usage: Assessing the amount of memory consumed by algorithms during execution.
- Big O Notation Analysis: Assigning algorithmic complexity based on their best, average, and worst-case scenarios.
Coding and Description:
- Code Structure and Logic: Implementing each algorithm with clear explanations of the logic and structure behind the code.
- Testing and Debugging: Rigorous testing to ensure the accuracy and reliability of implemented algorithms.
- Example Visualization:
Python Implementation of Bubble Sort
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Test case
array_to_sort = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(array_to_sort)
print("Sorted array:", sorted_array)
Results and Analysis
Presentation and Comparative Analysis:
- Visual Representations: Using tables, graphs, or visualizations to present experimental data for effective comparison.
- Algorithm Performance Evaluation: Analyzing and comparing the performance of different sorting algorithms based on the established metrics.
- Example Table:
This section will interpret the results garnered from the analysis. It will offer valuable insights derived from the experimental findings, highlighting the significance of algorithm efficiency in real-world applications. Limitations and challenges encountered during the study will be candidly discussed, shedding light on potential areas for improvement. Additionally, suggestions for further research and avenues to enhance algorithm efficiency will be explored, encouraging future investigations in this domain.
Summarizing the comprehensive exploration, the conclusion will encapsulate the key findings derived from the coursework's analysis. Emphasizing the importance of understanding and employing efficient algorithms in the realm of computer science, this section will reiterate the coursework's contributions to the broader understanding of algorithm efficiency. It will culminate with closing remarks that underscore the coursework's impact and potential implications in real-world applications.
References
2. Algorithms by Robert Sedgewick and Kevin Wayne
3. ACM Transactions on Algorithms (TALG)
4. IEEE Transactions on Computers
5. GeeksforGeeks Sorting Algorithms Articles
9. Coursera - Algorithms Specialization
10. MIT OpenCourseWare - Introduction to Algorithms
11. GitHub Repositories - Search for sorting algorithm implementations in various programming languages.
12. Stack Overflow - A community-driven platform for discussions on programming and algorithm implementations.